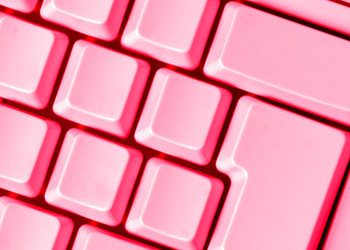
I was packing my apartment recently and found the notebook I used when interviewing for front end engineering positions last year. Below is a list of questions I was asked throughout the interview process.
JavaScript
I wrote a few blog posts about the following JavaScript topics, which you should understand if interviewing for front end roles: objects (including object literal notation and constructors), prototypes, declaration, initialization, hoisting, closures and array methods (including what the method returns, and whether it modifies the original array):
JavaScript Objects & Prototypes
JavaScript Variables: Declaration, Initialization, Hoisting & Closures
Common JavaScript Array Methods
What is the difference between == and === ?
== compares the value; === compares the value and the type:
"5" == 5; // true "5" === 5; // false
Explain the difference between null and undefined.
null is an object that has no value.undefined is a type.
Are you familiar with Jasmine or QUnit?
Jasmine and QUnit are JavaScript testing frameworks. I would familiarize yourself with the basics.
How would you call a function directly on a string?
See section on using prototypes to call functions on common data types in JavaScript Objects & Prototypes.
Explain event delegation.
Event delegation allows you to avoid adding event listeners for specific nodes. Instead, you can add a single event listener to a parent element. Here’s an example.
What is an IIFE?
IIFE stands for immediately-invoked function expression; it executes immediately after created by adding a () after the function.
What is an anonymous function?
Anonymous functions are functions without a name. They are stored in a variable and are automatically invoked (called) using the variable name.
var x = function(a, b) { console.log(a * b) } x(3, 5); // 15
Explain the difference between a host object and a native object.
Native – existing in JavaScript. Host – existing in the environment.
Explain the difference between call and apply.
apply lets you invoke the function with arguments as an array. call requires the parameters to be listed explicitly. Also, check out this stackoverflow answer.
Explain bind.
Check out this stackoverflow answer on the topic.
Explain variable scope.
JavaScript variables have functional scope. Here’s an article on the topic.
Explain this and that.
this and that are important to variable scope in JavaScript. Here are a few stackoverflow posts on this, that and self.
What is the difference between JSON and JSONP?
JSONP is JSON with padding. Here’s a good stackoverflow answer on the topic.
What is AJAX? Write an AJAX call.
AJAX stands for asynchronous JavaScript and XML and allows applications to send and retrieve data to/from a server asynchronously (in the background) without refreshing the page. For example, your new Gmail messages appear and are marked as new even if you have not refreshed the page.
What is a callback function?
JavaScript is read line by line. Sometimes, this can result in what seems like a subsequent line of code being executed prior to an earlier line of code. A callback function is used to prevent this from happening, because it is not called until the previous line of code has fully executed.
Here are two decent resources on callbacks with JavaScript and jQuery.
What is stringify?
stringify is used to transform JSON into a string.
What is the difference between a property and an attribute?
This blog post explains it.
What is the difference between a prototype and a class?
Prototype-based inheritance allows you to create new objects with a single operator; class-based inheritance allows you to create new objects through instantiation. Prototypes are more concrete than classes, as they are examples of objects rather than descriptions of format and instantiation.
Prototypes are important in JavaScript because JavaScript does not have classical inheritance based on classes; all inheritances happen through prototypes. If the JavaScript runtime can’t find an object’s property, it looks to the object’s prototype, and continues up the prototype chain until the property is found.
CSS
What is a float?
Floats are used to push elements to the left or right, so other elements wrap around it.
What is a clear?
A clear is used when you don’t want an element to wrap around another element, such as a float.
Have you ever used a CSS preprocessor/precompiler? What are the benefits?
CSS preprocessors, such as SASS, have numerous benefits, such as variables and nesting. For more information on preprocessors such as SASS, read my post Styling with SASS and Compass.
How do browsers read CSS?
From right to left.
What is Flexbox?
Here’s a great CSS Tricks guide to Flexbox.
Explain the difference between inline, block, inline-block and box-sizing.
inline is the default. An example of an inline element is <span>.
block displays as a block element, such as <div> or <p>.
inline-block displays an element as an inline-level block container. Here’s an article on the topic.
box-sizing tells the browser sizing properties. Here’s a CSS Tricks post on box-sizing.
Explain the difference between static, fixed, absolute and relative positioning.
static is the default.
fixed is positioned relative to the browser.
absolute is positioned relative to its parent or ancestor element.
relative is positioned relative to normal positioning/the item itself. Used alone it accomplishes nothing.
What is the difference between display:none and visibility:hidden?
Both will hide an element. However, display: none will render the page as if the element didn’t exist, while visibility: hidden will preserve the layout of the page as if the element were not hidden by leaving a space where the element would be.
What is the difference between responsive and adaptive development?
In a nutshell, responsive is fluid and flexible, whereas adaptive adapts to the detected device/screen size.
Other
Explain the difference between GET and POST.
A GET request is typically used for things like AJAX calls to an API (insignificant changes), whereas a POST request is typically used to store data in a database or submit data via a form (significant changes). GET requests are less secure and can be seen by the user in the URL, whereas POST requests are processed in two steps and are not seen by the user. Therefore, POST requests are more secure.
How can you increase page performance?
(1) Sprites, compressed images, smaller images; (2) include JavaScript at the bottom of the page; (3) minify or concatenate your CSS and JavaScript; and (4) caching.
Did I miss anything? If so, feel free to add to this post in the comments below.
- PM Career Story - April 28, 2022
- How to Transition into Product Management - December 26, 2017
- What I’ve Learned in My First Few Months as a Product Manager - October 14, 2015
Fantastic review of JS topics! Definitely a useful refresher for me.
FYI I assume you meant to write that CSS is right to left not left to right. Right? http://thenextweb.com/2011/03/22/writing-efficient-css-understand-your-selectors/
YES, good catch! Thanks, Ariel!
You’ve captured this petlfcrey. Thanks for taking the time!
Great resource, Koren. Thank you.
I would like to know you prepare for the interview by reading what books/websites?
Also, when you started out learning WordPress, what resources did you use in the first 2 weeks, before you can build WordPress sites/templates on your own?
Thank you for this post, and I wish you
I started learning WordPress years ago, but I could not build a theme from scratch. Re interviews, just google interview questions and practice using the concepts by building something. Good luck!
God, I feel like I shluod be takin notes! Great work
All things coindderes, this is a first class post
Great CSS writeup.