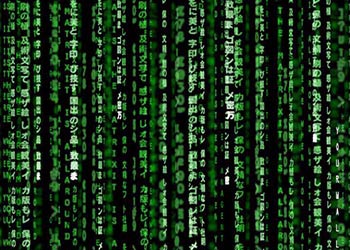
Takes user input and encrypts it with a key.
/** * caesar.c * * Koren Leslie Cohen * * Takes user input and encrypts it. * * Usage: ./asciimath key */ #include <stdio.h> #include <cs50.h> #include <stdlib.h> #include <string.h> #include <ctype.h> // encrypt user's word by number in command line int main(int argc, string argv[]) { // declare variables int key; int result; // only accept two command line arguments - key, plaintext string if (argc != 2) { printf("You didn't enter a key. Run program and enter key.\n"); return 1; } // get the plain text string PlainText = GetString(); // convert the string/second command line argument (number) to integer key = atoi(argv[1]); // if key >= 26, use modulo 26 to wrap back to Aa after Za if (key >= 26) { key = (key % 26); } // encrypt - iterate over characters in string // print each one one at a time for(int i = 0, length = strlen(PlainText); i < length; i++) { // test - printf("In calculating %c + %d...\n", PlainText[i], key); // encryption result = (PlainText[i] + key); // wrapping after Z for uppercase letters if (isupper(PlainText[i]) && (result > 'Z')) { result = (result - 26); } // wrapping after z for lowercase letters if (islower(PlainText[i]) && (result > 'z')) { result = (result - 26); } // test - printf("The ASCII value of %c is %d.\n\n", result, result); // if character is alphabetical, print encrypted result if (isalpha(PlainText[i])) { printf("%c", result); } // if non-alphabetical character, print as is else { printf("%c", PlainText[i]); } } printf("\n"); return 0; }
Result:
Latest posts by Koren Leslie Cohen (see all)
- PM Career Story - April 28, 2022
- How to Transition into Product Management - December 26, 2017
- What I’ve Learned in My First Few Months as a Product Manager - October 14, 2015
With a little added coindg you can specify the number of decimal places, as well as deal with negative numbers:function round(num,places, sign) { # Rounds to /places/ decimal points – if /places/ not supplied it is # treated as 1, also can supply negative /places/ places=10^places sign=1 if (num < 0) {sign = -1; num = -num;} return sign * int(num*places + .5)/places}
Thanks!
Thank you … 🙂 It helped me a lot…!!
thanks.. 🙂 <3
I am trying to complete Caesar Cipher. I don’t understand your algorithm. Can you be a little more descriptive?
Message me directly.
Your code really helped me with the cs50 edx course I’m taking. Thank you for taking the time to post all your solutions.
Very elegant Algorithm. Thank you for posting it! Helped me to implement it in C!
Greetings from Germany!